Пример: С помощью FTP доступа, соединится с удаленным сервером, создать папку и сделать листинг папок в существующей папке.
Текст программы:
<?php
Class FTPClient
{
private $connectionId;
private $loginOk = false;
private $messageArray = array();
/////////////////////////////////////////////////////
public function __construct() { }
/////////////////////////////////////////////////////
private function logMessage($message)
{
$this->messageArray[] = $message;
}
/////////////////////////////////////////////////////
public function getMessages()
{
return $this->messageArray;
}
/////////////////////////////////////////////////////
public function connect ($server, $ftpUser, $ftpPassword, $isPassive = false)
{
// *** Set up basic connection
$this->connectionId = ftp_connect($server);
// *** Login with username and password
$loginResult = ftp_login($this->connectionId, $ftpUser, $ftpPassword);
// *** Sets passive mode on/off (default off)
ftp_pasv($this->connectionId, $isPassive);
// *** Check connection
if ((!$this->connectionId) || (!$loginResult)) {
$this->logMessage('FTP connection has failed!');
$this->logMessage('Attempted to connect to ' . $server . ' for user ' . $ftpUser, true);
return false;
} else {
$this->logMessage('Connected to ' . $server . ', for user ' . $ftpUser);
$this->loginOk = true;
return true;
}
}
/////////////////////////////////////////////////////
public function changeDir($directory)
{
if (ftp_chdir($this->connectionId, $directory)) {
$this->logMessage('Current directory is now: ' . ftp_pwd($this->connectionId));
return true;
} else {
$this->logMessage('Couldn\'t change directory');
return false;
}
}
/////////////////////////////////////////////////////
public function getDirListing()
{
$parameters = '-la';
$directory = '.';
// get contents of the current directory
// получить содержимое текущей директории
$contents = ftp_nlist($this->connectionId, ".");
// вывод $contents
// var_dump($contents);
print_r($contents);
}
/////////////////////////////////////////////////////
public function makeDir($directory)
{
// *** If creating a directory is successful...
if (ftp_mkdir($this->connectionId, $directory)) {
$this->logMessage('Directory "' . $directory . '" created successfully');
return true;
} else {
// *** ...Else, FAIL.
$this->logMessage('Failed creating directory "' . $directory . '"');
return false;
}
}
/////////////////////////////////////////////////////
public function __deconstruct()
{
if ($this->connectionId) {
ftp_close($this->connectionId);
}
}
}
// *** Впишите сюда ваш хост, имя пользователя и пароль
define('FTP_HOST', '178.170.251.135');
define('FTP_USER', 'laba9');
define('FTP_PASS', 'RfP6yrar');
// *** Create the FTP object
$ftpObj = new FTPClient();
// *** Connect
$ftpObj -> connect(FTP_HOST, FTP_USER, FTP_PASS);
// *** Connect
echo "<p>";
if ($ftpObj -> connect(FTP_HOST, FTP_USER, FTP_PASS)) {
// *** Then add FTP code here
echo 'ФТП Подключен';
} else {
echo 'ФТП Не удалось подключиться ';
}
echo "<p>";
$dir = 'photos';
// *** Make directory
$ftpObj->makeDir($dir);
$ftpObj->changeDir($dir);
$ftpObj->makeDir('papka1');
$ftpObj->makeDir('papka2');
$ftpObj->makeDir('papka3');
// *** Get folder contents
echo "<p>";
print_r($ftpObj -> getMessages());
echo "<p>";
$contentsArray = $ftpObj->getDirListing();
?>
Результат работы программы:
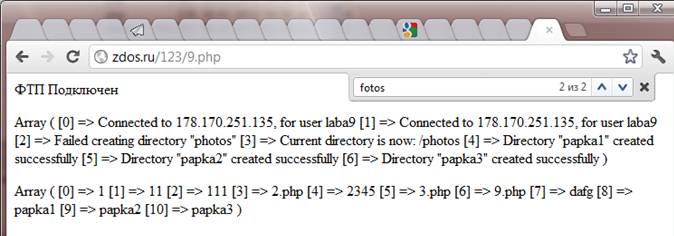